Project One – Blog Template
We will be building a blog template. The final output will be as shown below:
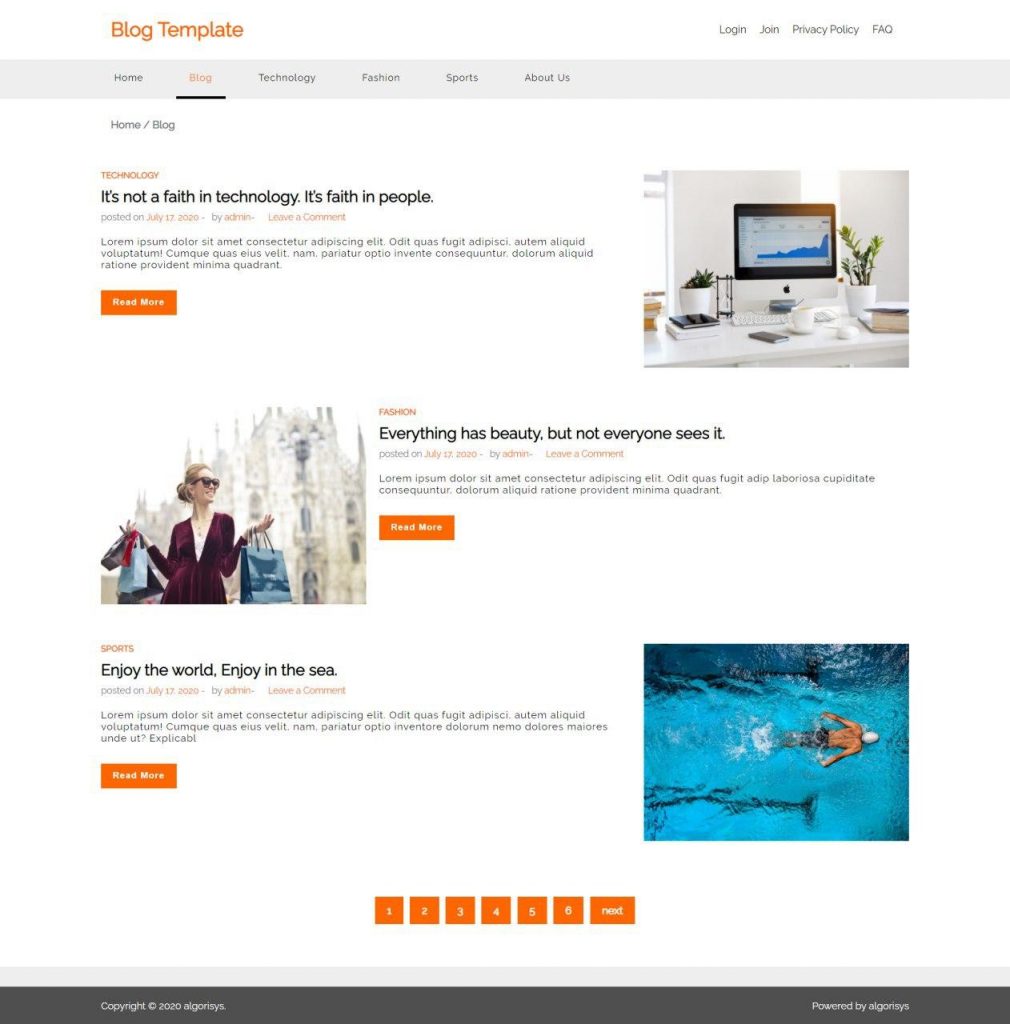
We will be creating a blog by making use of the CSS you have learned so far.
Let’s create the HTML using header, nav, section, div, and footer and
a few more with an external style sheet named ‘style.css’.
<!DOCTYPE html>
<html>
<!-- Below the the head tag, where the title, the meta details,
the styles and other links go -->
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Blog Template</title>
<link rel="stylesheet" type="text/css" href="css/style.css">
</head>
<body>
<header class="header">
<div class="container">
<div class="header-top d-flex">
<div class="top-left">
<a class="logo show-lg" href="#">Blog Template</a>
<a class="logo show-sm" href="#">BT</a>
</div>
<div class="top-right">
<ul class="right-nav">
<li><a href="#">login</a></li>
<li><a href="#">join</a></li>
<li><a href="#">privacy policy</a></li>
<li><a href="#" style="text-transform: uppercase;" href="#">faq</a></li>
</ul>
</div>
</div>
</div>
<!-- Here in nav tags, we provide all the links to other pages -->
<nav class="nav">
<button onclick="toggleButton()" class="toggle">
<div></div>
<div></div>
<div></div>
</button>
<div class="container">
<ul id="navigation" class="navbar">
<li>
<a href="#">home</a>
</li>
<li>
<a class="active" href="#">blog</a>
</li>
<li>
<a href="#">Technology</a>
</li>
<li>
<a href="#">Fashion</a>
</li>
<li>
<a href="#">Sports</a>
</li>
<li>
<a href="#">about us</a>
</li>
</ul>
</div>
</nav>
</header>
Lets add some content which goes in side the body.
<!-- Start of the body content - give it an id of main-page -->
<div class="container" id="main-page">
<!-- Add Breadcrumbs -->
<div class="breadcrumbs">
<h4>home / <span> blog </span></h4>
</div>
Now, add the first section of the blog using the <section >tag.
<!-- Section One -->
<section>
<img src="https://images.pexels.com/photos/572056/pexels-photo-572056.jpeg">
<div class="section-info">
<div>
<a href="#">TECHNOLOGY</a>
</div>
<h2>It’s not a faith in technology. It’s faith in people.</h2>
<ul class="blog-detail">
<li class="posted-date">posted on <a href="#"> July 17, 2020 </a>-
</li>
<li class="by-admin">by<a href="#"> admin</a>-
</li>
<li class="comment">
<a href="#">Leave a Comment</a>
</li>
</ul>
<p>
Lorem ipsum n pariatuquadrant.mnnlkkjjk k kj
</p>
<button class="read-button">read more</button>
</div>
</section>
Similarly, create two more sections with different contents.
<!-- Section Two -->
<section >
<img src="https://images.pexels.com/photos/972995/pexels-photo-972995.jpeg">
<div class="section-info">
<div><a href="#">FASHION</a></div>
<h2>Everything has beauty, but not everyone sees it.</h2>
<ul class="blog-detail">
<li class="posted-date">posted on <a href="#"> July 17, 2020 </a>-
</li>
<li class="by-admin">by<a href="#"> admin</a>-
</li>
<li class="comment"><a href="#">Leave a Comment</a>
</li>
</ul>
<p>
Lorem ipsum dolor sit amet consectetur adipiscing elit. Odit quas fugit ias non</p>
<p>
Lorem ipsum dolor sit amet consectetur adipiscing elit. Odit quas fugit ias non</p>
<button class="read-button">read more</button>
</div>
</section>
<!-- Section Three -->
<section class="">
<img src="https://images.pexels.com/photos/863988/pexels-photo-863988.jpeg">
<div class="section-info">
<div><a href="#">SPORTS</a></div>
<h2>Enjoy the world, Enjoy in the sea.</h2>
<ul class="blog-detail">
<li class="posted-date">posted on <a href="#"> July 17, 2020 </a>-
</li>
<li class="by-admin">by<a href="#"> admin</a>-
</li>
<li class="comment"><a href="#">Leave a Comment</a>
</li>
</ul>
<p>
Lorem ma quadrant. dfgd gffgdfg fg fg fg
</p>
<button class="read-button">read more</button>
</div>
</section>
Let’s create a pagination link.
<div class="pagination-wrapper">
<ul class="pagination">
<li><a href="#">1</a></li>
<li><a href="#">2</a></li>
<li><a href="#">3</a></li>
<li><a href="#">4</a></li>
<li><a href="#">5</a></li>
<li><a href="#">6</a></li>
<li><a href="#">next</a></li>
</ul>
</div>
Lastly, lets create the footer.
<footer class="footer">
<div class="footer-top">
<div class="footer-left">
<p>Copyright © 2020 algorisys.</p>
</div>
<div class="footer-right">
<p>Powered by algorisys</p>
</div>
</div>
</footer>
Below, we will add the end part of the template where we are using some javascript code for the responsive navigation menu. You can learn more about javascript here. Learn JavaScript
<!-- javascript code for menu -->
<script type="text/javascript">
function toggleButton() {
var x = document.getElementById("navigation");
if (x.style.display === "block") {
x.style.display = "none";
} else {
x.style.display = "block";
}
}
</script>
</body>
</html>
The CSS styles are given below.
* {
margin: 0;
padding: 0;
}
body {
font-size: 16px;
font-family: 'Raleway', sans-serif;
}
a {
text-decoration: none;
}
ul {
list-style-type: none;
}
.container {
display: block;
width: 100%;
margin: 0 auto;
clear: both;
}
.d-flex {
display: flex;
}
.show-sm {
display: block;
}
.show-lg {
display: none;
}
.header {
width: 100%;
height: auto;
display: block;
overflow: hidden;
}
.logo {
font-size: 30px;
font-weight: bold;
text-transform: capitalize;
color: #ff6600;
}
.header-top {
height: 70px;
align-items: center;
margin: 10px auto;
display: flex;
justify-content: space-between;
padding: 0px 15px;
}
.right-nav {
list-style: none;
}
.right-nav li {
padding-left: 10px;
padding-right: 10px;
float: left;
}
.right-nav li a {
font-size: 16px;
text-transform: capitalize;
font-weight: 500;
color: #000;
cursor: pointer;
}
.right-nav li a:hover {
color: #ff6600;
}
.nav {
background-color: #EEEEEE;
display: block;
overflow: hidden;
}
.navbar {
margin: 0 auto;
display: block;
flex-wrap: wrap;
justify-content: center;
}
.navbar li {
border-bottom: 2px solid #fff;
}
.navbar li:first-child {
border-top: 2px solid #fff;
}
.navbar li a {
font-size: 14px;
color: #000;
font-weight: 500;
letter-spacing: 1.2px;
text-transform: capitalize;
padding: 10px 20px;
display: block;
border-bottom: 4px solid transparent;
margin: 0 auto;
text-align: center;
cursor: pointer;
}
.navbar li a:hover {
color: #fff;
background-color: #FF6600;
}
.navbar li a.active {
color: #fff;
background-color: #FF6600;
}
.toggle {
padding: 5px 10px;
background-color: transparent;
border: 1px solid #ff6600;
font-size: 18px;
text-transform: capitalize;
font-weight: 600;
display: block;
margin: 10px 15px;
float: right;
overflow: hidden;
cursor: pointer;
}
.toggle div {
width: 30px;
height: 2px;
background-color: black;
margin: 6px 0;
}
#navigation {
display: none;
}
#main-page {
height: auto;
margin: auto;
overflow-x: hidden;
}
.breadcrumbs {
display: block;
overflow: hidden;
margin: 30px auto 15px;
padding: 0px 15px;
}
.breadcrumbs h4 {
font-size: 16px;
font-weight: 600;
color: #75777d;
text-transform: capitalize;
}
section {
height: auto;
margin: 60px auto;
display: block;
overflow: hidden;
padding: 0px 15px;
}
section img {
height: 255px;
margin: 0 auto;
flex: 1;
display: block;
overflow: hidden;
}
section:nth-child(odd) img {
padding-right: 20px;
}
section:nth-child(even) img {
order: 2;
}
.section-info {
flex: 2;
height: auto;
display: block;
margin-top: 30px;
}
.section-info div a {
color: #ff6600;
margin-bottom: 10px;
font-size: 13px;
font-weight: bold;
display: block;
}
.section-info div a:hover {
text-decoration: underline;
}
.section-info p {
margin-top: 20px;
letter-spacing: 1px;
font-size: 15px;
}
.blog-detail {
list-style: none;
margin-top: 10px;
margin-bottom: 10px;
display: block;
overflow: hidden;
}
.blog-detail li {
float: left;
color: #75777d;
font-size: 14px;
}
.posted-date {
padding-right: 10px;
}
.by-admin {
padding-right: 10px;
}
.comment {
padding-left: 10px;
}
.blog-detail li a {
color: #ff6600;
}
.read-button {
padding: 10px 17px;
color: #ffffff;
letter-spacing: 1.2px;
background-color: #ff6600;
border: 1px solid #ff6600;
text-transform: capitalize;
margin-top: 30px;
font-weight: 600;
}
.pagination-wrapper {
width: 100%;
height: auto;
margin: 80px auto 20px auto;
display: block;
overflow: hidden;
}
.pagination {
width: 80%;
height: auto;
display: flex;
justify-content: center;
margin: 0 auto;
}
.pagination li {
padding: 5px;
}
.pagination li a {
background-color: #ff6600;
border: 1px solid #ff6600;
padding: 10px 17px;
display: block;
color: #ffffff;
font-weight: 600;
}
.footer {
width: 100%;
height: auto;
overflow: hidden;
background-color: #000000b0;
border-top: 30px solid #EEEEEE;
display: block;
margin: auto;
margin-top: 40px;
}
.footer-top {
width: 80%;
height: auto;
margin: 0 auto;
display: flex;
justify-content: space-between;
padding: 20px;
}
.footer-left {
color: #ffffff;
font-size: 15px;
font-weight: 500;
}
.footer-right {
color: #ffffff;
font-size: 15px;
font-weight: 500;
}
To make the above site responsive, we need to add some media queries in our CSS for the site. This will create a responsive site with a responsive menu for the smaller screens.
/***media query***/
@media screen and (min-width: 768px) {
.show-sm {
display: none;
}
.show-lg {
display: block;
}
.container {
display: block;
width: 80%;
margin: 0 auto;
clear: both;
}
.navbar {
margin: 0 auto;
display: block;
}
.navbar li {
float: left;
margin-left: 15px;
margin-right: 15px;
border-bottom: none;
}
.navbar li:first-child {
margin-left: 0px;
border-top: none;
}
.navbar li a {
font-size: 14px;
padding: 20px;
}
.navbar li a:hover {
color: #ff6600;
border-bottom: 4px solid #000;
background-color: transparent;
}
.navbar li a.active {
color: #ff6600;
border-bottom: 4px solid #000;
background-color: transparent;
}
.toggle {
display: none;
}
#navigation {
display: block;
}
section {
display: flex;
padding: 0px;
}
.section-info {
margin-top: 0px;
}
section img {
height: 300px;
margin: 0 0;
}
section:nth-child(even) img {
order: 2;
padding-left: 20px;
}
}
We are done with the blog template. You can check the final result in the browser.
The source code of the blog can be downloaded below.